Definition of Flutter appbar widget, its usage, importance in a Flutter app, syntax of appbar will be explained. Its constructors will be explained practically with 14 proper and easy code examples.
What is Flutter Appbar Widget?
Flutter appbar is a bar that can be shown in the top position of Flutter app. We can put drawer icons, back icons, title, other action buttons like logout, menu and many more. It makes the Flutter app very attractive and allows the users to use app with ease.
Syntax of appbar in Flutter
Scaffold( appBar: AppBar() )
Constructors of Flutter Appbar
The list is:
- background color
- title
- center title
- title spacing
- title text style
- actions
- actions icon theme
- leading
- automatically imply leading
- leading width
- elevation and shadow color
- flexible space
- toolbar height
- bottom
- bottomOpacity
Example 1: background color of app bar
backgroundColor: Colors.green
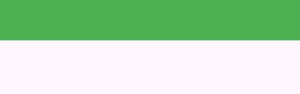
Example 2: title of appbar
title: Text('Appbar Title')
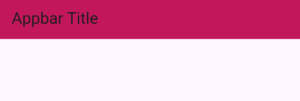
Example 3: center title
centerTitle: true
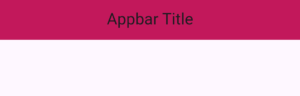
This constructor takes a boolean value. It’s role is to place the title in the center of appbar. By default, its false, so making it true placed the title in center as shown in the above image.
Example 4: title spacing from left
titleSpacing: 0
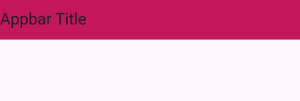
titleSpacing: 41
Example 5: title text style
titleTextStyle: TextStyle( color: Colors.white, fontSize: 19, fontWeight: FontWeight.bold)
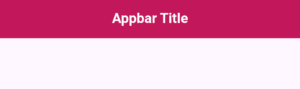
Example 6: actions constructor
actions: [ Icon(Icons.logout), IconButton(onPressed: () {}, icon: Icon(Icons.person),), ],

Example 7: actions icon theme
actionsIconTheme: IconThemeData(color: Colors.white,size: 21,)
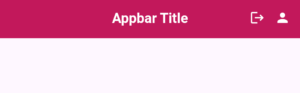
Example 8: leading constructor
leading: TextButton( onPressed: (){ Navigator.of(context).pop(); }, child: Text('Back'))
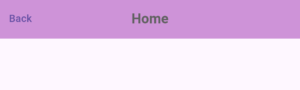
Example 9: automatically imply leading
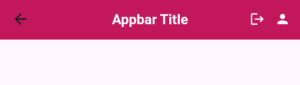
automaticallyImplyLeading: false
Example 10: leading width
leadingWidth: 120

Example 11: elevation and shadow color
elevation: 5, shadowColor: Colors.black

- The elevation gives the appbar a shadow effect so it looks elevated from the screen. With it we have to set some color to the shadowColor constructor as well or else we won’t see any shadow.
- The elevation takes a double but you can pass integer as well and the shadow color takes a color.
- You can pass the color code as well as I have explained in the appbar background color section of this article.
- The more elevation value, the more shadow it will spread. You can play by modifying the values until you reached your desired design.
Example 12: flexible space
flexibleSpace: Container( color: Colors.pink.shade700, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Home', ), Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: [ Text( 'Home again 1', ), Text( 'Home again2', ), ], ), ], ), ),
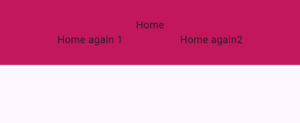
Example 13: toolbar height
toolbarHeight: 180
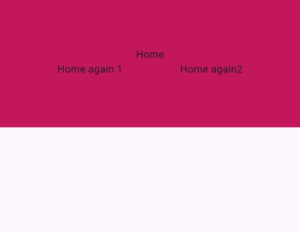
Example 14: bottom constructor for Flutter Appbar tabs
Sometimes we need to use tabs in the appbar to navigate/shift to screens. For that, we use the bottom constructor.
It shows the item at the bottom of Flutter appbar. Actually, we can use it to show tabbar. See below code:
DefaultTabController( length: 3, initialIndex: 0, child: Scaffold( appBar: AppBar( bottom: TabBar( tabs: [ Tab(icon: Icon(Icons.home), text: 'Home'), Tab(icon: Icon(Icons.search), text: 'Search'), Tab(icon: Icon(Icons.person), text: 'Profile'), ], ), centerTitle: true, backgroundColor: Colors.purple.shade100, toolbarHeight: 80, title: Text( 'Home', style: TextStyle( color: Colors.grey.shade700, fontSize: 19, fontWeight: FontWeight.bold), ), ), body: TabBarView( children: [ Center(child: Text('Home Page')), Center(child: Text('Search Page')), Center(child: Text('Profile Page')), ], ), ), ),
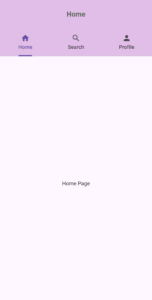
- First I wrapped our scaffold widget with default tab controller and assigns the number to 3. You can specify 4 or more/less as well but make sure that the number of tabs matches everywhere.
- Then I passed the tab bar widget to the bottom constructor. By passing a list of tabs to the tabs constructor, I specified tabs in our Flutter appbar widget.
- In body, I passed the tab bar view widget to show the screens depending on the selected tab. It’s order-sensitive so the first item will be assigned to the first tab, the second child to the second tab, and so on. You can pass any widgets you want to the list passed to the tab bar view but make sure that the numbers of tab bar view and tab view items are same.
- In our later article, I will explain the bottom and tabs of appbar widget in more detail.
Example 15: bottomOpacity of Flutter Appbar
bottomOpacity: 0.4
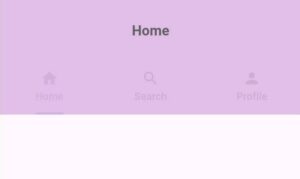
- The bottomOpacity parameter of Flutter appbar is used to decide how much visible the bottom of appbar should be.
- It’s default value is 1.0. It takes a double value from 0 to 1. The lesser the value is from 1 the less visible the bottom will be.
- To demonstrate it, we passed it a value 0.4 and you can see that the bottom tabs are less visible now. If you pass it 0 then they won’t be visible but still if you tap on them then they will change tabs as before.
- It means the bottomOpacity of appbar just modify the visibility of the appbar bottom.
Conclusion
I hope you now have a detailed understanding of how to properly customize and use Flutter appbar widget. Do comment if you have any problems/queries with appbar widget, I will be happy to solve them.
Thank you.